Getting Started with Leiapix API
LeiaPix APIs offer developers a seamless and user-friendly experience through a set of powerful RESTful APIs. Harness the capabilities of our APIs to effortlessly generate disparity maps from 2D images and unlock the potential for creating breathtaking 3D animations from your 2D image collection.
OpenAPI
LeiaPix API is described in accordance with the OpenAPI v3.0 Specification (OAS).
This allows developers to easily integrate their programs or scripts with LeiaPix API.
Instead of manually writing the API client code for each endpoint, a very common practice is to automatically generate it. For the most languages, there are available libraries, plugins and CLI tools that take the OAS file or URL as input and generate a fully usable client code.
The latest LeiaPix OAS is available here.
π Authentication
In order to access our APIs, you will need a Leia Account. If you do not already have one, you can easily create on LeiaPix. You can then manage your client ID and secrets in the Leia Account Page.
Getting Your Client ID and Secret
LeiaPix APIs uses Client Credentials flow for providing access to the resources. Once you create and login with your account, click on Leia Account on the top right hand corner of the window.
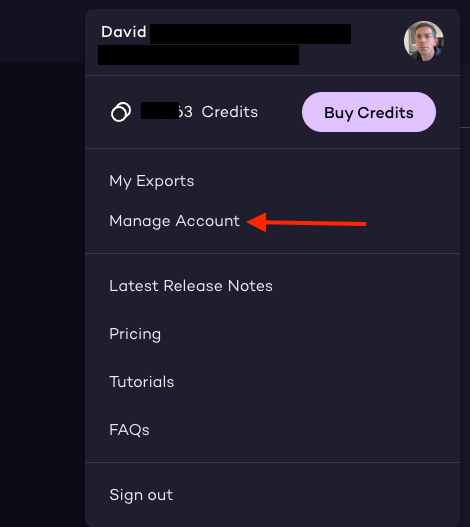
Click on API tab. This is where you can manage all your Client ID and Secret Key pairs.
Click on β+β sign to add a new Client ID and Secret Key Pair.
Your Client credentials, client IDs and secrets, should be treated as sensitive information. Anyone getting access to these can drain your credits by using these secrets and keys. You should never share or expose your client credentials publicly. A few additional approaches include:
Use Secure Storage: Store your client credentials and secrets securely. It is recommended to use encrypted storage or a secure key management system to safeguard this information.
Avoid Embedding Credentials in Code: Avoid hard-coding client credentials directly in application code or configuration files. Instead, consider using environment variables or configuration files separate from the application code.
Regularly Rotate Secrets: It is a good idea to periodically rotate client secrets and credentials to mitigate the risk of unauthorized access. This practice adds an extra layer of security and helps prevent potential security breaches.
Exchanging your Client ID and Secret for an Access Token
In order to obtain an access token with your client credentials, use the Get Access Token API endpoint.
LeiaPix API Uses OAuth2 client credentials flow. Please refer to OAuth2 documentation for more details about this authentication flow.
π Credits
Credits are required to use LeiaPix API.
Credits are the currency used to access and utilize the features of our API and in Leia Applications.
Each API request consumes a certain amount of credits based on its complexity and resources utilized. To make API requests, you need to have a sufficient number of credits in your account.
As a promotional offer, each new account will initially receive 100 free credits and each API will require a flat rate of 10 credits per call. Currently, you can also use these credits with LeiaPix API or LeiaPix.com.. However, free credits can't be used for commercial purposes. After depleting your free credits, additional credits can be purchased via your account.
Click on your profile picture on Leiapix, then click Buy Credits.
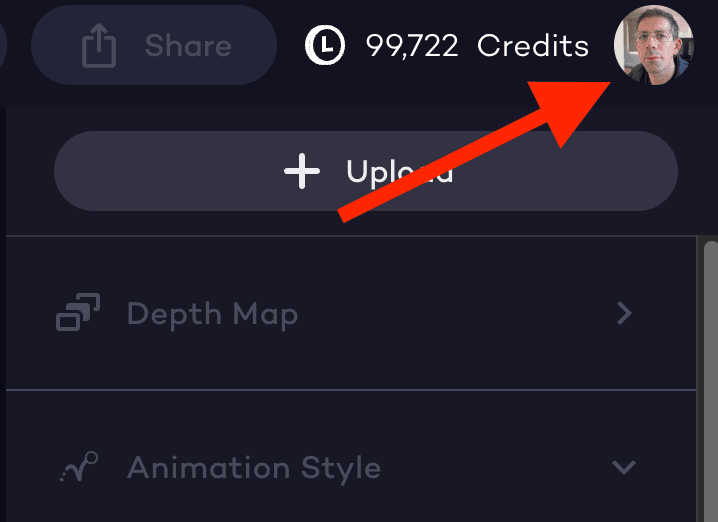
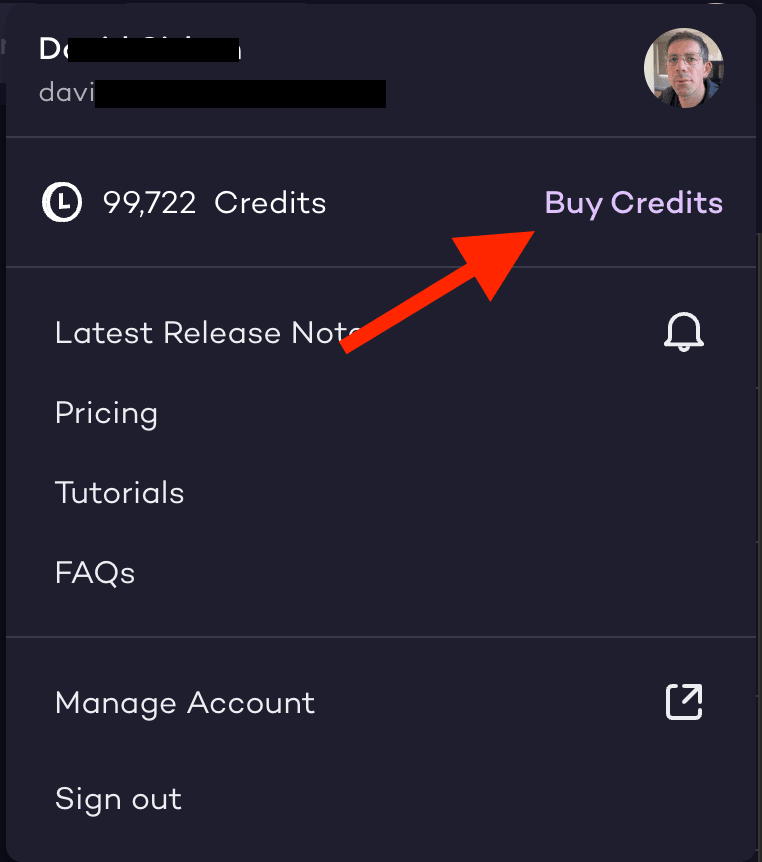
Once you make the payment, Credits will be reflected in your account.
Pre-Signed URLs
LeiaPix APIs use pre-signed URLs to write the output data to. This approach provides, among other things,
Reduced Server Load: Offloading the data upload process to the client and storage provider reduces the workload on the API server. This allows us to handle more concurrent requests and improves overall API performance.
Scalability: By leveraging the scalability of cloud storage providers, we can accommodate large-scale data uploads without straining server resources.
Faster Uploads: With direct client-to-storage uploads, data transfer is more efficient, leading to faster upload times compared to having the data pass through multiple servers.
Simplified Error Handling: The direct upload approach simplifies error handling since most issues, such as network interruptions or upload failures, are handled by the storage provider, and the client can be notified accordingly.
These URLs must have HTTP PUT permissions embedded. If you would like to learn more about the pre-signed URLs, please refer to your respective cloud provider.
Azure Blob Storage Shared Access Signature (SAS)
API Usage Example snippets
Python:
import uuid
import requests
LEIA_LOGIN_OPENID_TOKEN_URL = (
"https://auth.leialoft.com/auth/realms/leialoft/protocol/openid-connect/token"
)
correlation_id = str(uuid.uuid4())
token_response = requests.post(
LEIA_LOGIN_OPENID_TOKEN_URL,
data={
"client_id": YOUR_CLIENT_ID,
"client_secret": YOUR_CLIENT_SECRET,
"grant_type": "client_credentials",
},
).json()
access_token = token_response["access_token"]
response = requests.post(
"https://api.leiapix.com/api/v1/disparity",
headers={"Authorization": f"Bearer {access_token}"}, # Acquired in previous step
json={
"correlationId": correlation_id,
"inputImageUrl": ORIGINAL_IMAGE_URL, # Link to the image you want to get disparity for
"resultPresignedUrl": put_disparity_presigned_url, # Presigned URL with HTTP PUT permission.
},
timeout=5 * 60, # expires in 5 min
)
# Check if the response code is 2XX
Node.js
import axios from "axios";
import { v4 as uuidv4 } from "uuid";
const correlationId = uuidv4();
await axios.post(
"https://api.leiapix.com/api/v1/disparity",
{
correlationId,
inputImageUrl: ORIGINAL_IMAGE_URL, // URL to the image you want to create disparity map for
resultPresignedUrl: putDisparityPresignedUrl, // Presigned URL with HTTP PUT permission where disparity map will be created
},
{
headers: {
Authorization: `Bearer ${accessToken}`, // acquired in previous step
},
timeout: 3 * 60 * 1000, // request times out after 3 minutes
}
);
Sample
You can find a python and node.js sample here
π¬ We're here to help!
If you need any help, please join our Discord channel for support.
Discord Rules
- Be respectful and professional: Treat others with respect and maintain a professional tone in all discussions and interactions.
- Stay on topic: Keep conversations and questions related to our APIs, features and functionality. Off-topic discussions should be redirected or moved to the appropriate channels.
- Use appropriate language: Use polite and appropriate language at all times. Avoid offensive, derogatory, or inappropriate content.
- No spam or self-promotion: Do not spam the server with irrelevant content or promote personal products, services, or links without prior permission.
- Be patient and helpful: Be patient when assisting developers with their queries. Offer help and support in a constructive and friendly manner.
- Provide clear instructions: When seeking assistance, clearly explain the issue and provide relevant information such as code snippets, error messages, or steps to reproduce the problem.
- Search before asking: Before posting a question, search the server and read existing discussions to avoid duplicate topics. Utilize the available resources, such as documentation and FAQs, whenever possible.
- Respect privacy and confidentiality: Do not share sensitive information, such as API keys or personal data, in public channels. Use direct messaging or secure communication channels for such purposes.
- No harassment or bullying: Harassment, bullying, or any form of discriminatory behavior will not be tolerated. Treat all members with respect and kindness.
- Follow Discord's Terms of Service: Adhere to Discord's Terms of Service and Community Guidelines. Familiarize yourself with these guidelines and ensure your conduct aligns with them.
- Respect team decisions: The moderation team has the final say on any disputes or issues. Respect and follow the decisions made by the team.
- No adult content or gore. Please avoid making visually shocking or disturbing content. We will block some text inputs automatically.
- Do not publicly repost the creations of others without their permission.
- Be careful about sharing. Itβs OK to share your creations outside of the LeiaPix Cloud community, but please consider how others might view your content.
We're excited you're here! π
Updated 1 day ago